The Payfonte checkout page is a page that allows customers to complete their purchases. Here is how it works:
- From your infrastructure/application, call our Generate Checkout URL Endpoint with the payment details.
- We will provide you with a link to a payment page, which you can then redirect your customer to in order to complete the payment process.
- Once the transaction is complete, we will redirect the customer back to you.
1. Get all the payment details
First, you need to assemble the payment details. Here are the details you'll need:
Field | Type | Description | Required |
---|---|---|---|
amount | Number | This is the amount to charge the customer. This should be in the lowest currency denomination | True |
user | Object | This is an object containing the users email , phoneNumber and name | True |
user.email | String | This is the user's email address. | True |
user.phoneNumber | String | This is the user's phone number | False |
user.name | String | This is the user's name | False |
reference | String | A reference code you'll generate to identify this transaction. This must be unique for every transaction. If you don't pass one, we will generate one for you. | False |
currency | String | This specifies the currency in which you would like to charge. If you do not provide a value, we will assume "NGN". | True |
country | string | This specifies the country you are processing the transaction for, this also determines the integrations that ill be shown to the user | True |
redirectURL | String | The URL to which the customer should be redirected after the payment is completed. | False |
customerBearsCharge | Boolean | This determines if we are to shift the burden of paying the provider charge to the customer or not. Defaults to false | False |
webhook | String | This is a URL, if this is passed we will send the event webhook payload to this endpoint | False |
2. Call the endpoint to generate a checkout URL
Next, in order to initiate the payment process, you will need to call our Generate Checkout URL Endpoint API and provide the collected payment details. Please ensure that you authorize the API call using your client-id and client-secret. Below is an example in Node.js:
curl --location 'https://sandbox-api.Payfonte.io/payments/v1/checkouts' \
--header 'client-id: Payfonte' \
--header 'client-secret: dev_8334e61c10c81ab14a302376e7986683fb2c7d225db580fb64' \
--header 'Content-Type: application/json' \
--data-raw '{
"reference": "0f0d9d90-9099-435e-9aa1-2c000ef8598b",
"amount": 50000,
"redirectURL": "https://payfonte.com",
"customerBearsCharge": false,
"webhook": "https://webhook.site/93745537-9d53-4aeb-b8ac-a489e0dd9967",
"user": {
"email": "dummy@dummy.com",
"phoneNumber": "07012345678"
}
}'
After making the API call, you will receive a response similar to this:
{
"data": {
"id": "644ebe614c2604002fac9d13",
"url": "https://checkout-staging.payfonte.com/payfusion/644ebe614c2604002fac9d13",
"shortURL": "https://l.6bd.co/m_DbfggPw",
"reference": "0ba83bfd-9bba-48d4-b182-57cb4d1442cb",
"amount": 50000
}
}
3. Redirect the user to the generated payment link
Once you receive the response, simply redirect your customer to the link provided in data.shortURL
or data.url
. Our checkout modal will be displayed to your customer, allowing them to complete the payment process.
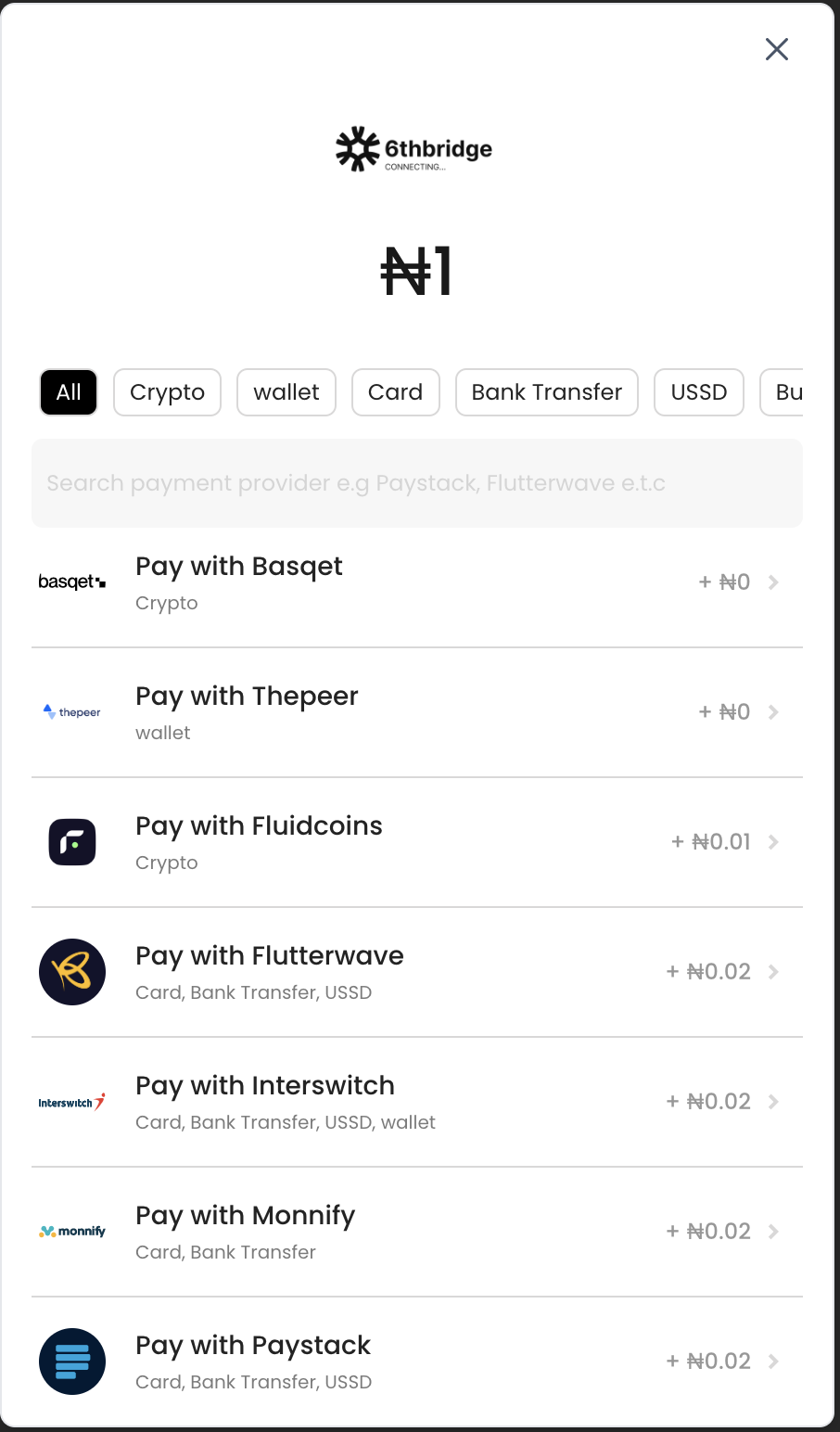
4. After the payment
This following event will occur after a payment is completed
- The customer will be redirected to the URL specified in
redirectURL
with thestatus
andreference
. - If you have webhooks enabled, we will send you a notification via webhook once the payment is completed. To learn more about webhooks and see examples, please visit the following link Webhooks.
5. When Payment Fails
In the event that a payment attempt fails, such as due to insufficient funds, there is no need for you to take any action. The payment page will remain open so that the customer can try again until the payment is successful or they choose to cancel.
If you have webhooks enabled, we will send you a notification for each failed payment attempt. This can be useful if you need to follow up with customers who had issues with payment at a later time. Please refer to our Webhooks guide for an example of how this works.